Inheritance and Types of Inheritance
1. Introduction
The concept inheritance mainly relies on reusability of software or application programs. That means C++ classes can be reused in various ways. We can create new classes from old classes without affecting the original one. The process of creating a new class from an existing class is called as inheritance.
2. Creating a Derived Class
The general form of creating a derived class is given as:
class derived_class_name:access_specifier base_class_name
{
//Members of derived class
};
Consider the following example:
Class B: public A
{
________
_________
};
Here, class B is a derived class, and A is a base class. Both are separated by a colon(:).
The access specifiers decide whether the characteristics of the base class inherited members as private, public or protected within the derived class.
If no access specifier is mentioned, then the default mode is private.
Example
Class B:A
{
// Inherited member of class A will become
// private in class B
};
3. Access Specifiers with their Scope
The members of a class under protected access specifier exhibit data hiding like that of private members of the class. But private members cannot be inherited, whereas protected members can be inherited to its derived class.
The following Table 6.1will help the reader to understand the access specifiers scope.
Table 1
Base
class Mode
|
Derived class Mode
|
private
|
public
|
protected
|
private
|
Not inherited
|
Not inherited
|
Not inherited
|
public
|
private
|
public
|
protected
|
protected
|
private
|
protected
|
protected
|
It is clear from the table that private members of a base class cannot be inherited, however protected and public members of a class can be inherited and their characteristics will be changed in derived class as per the mode(access specifier) specified during creation of derived class.
4. Types of Inheritance
The various types of inheritance are
§ Single Level Inheritance
§ Multilevel Inheritance
§ Multiple Inheritance
§ Hybrid Inheritance
§ Hierarchical Inheritance
4.1. Single Level Inheritance
When a single class(B) is derived from a single base class(A), then the inheritance is called as single level inheritance.
↓
Figure 1 Single Level Inheritance
Program 1
Write a program to implement single inheritance as per the structure given in figure 1.
#include<iostream.h>
#include<constream.h>
class A
{
protected:
int rollno;
char name[15];
};
class B:private A
{
private:
int mark1;
int mark2;
public:
void getdata()
{
cout<<"Enter rollno and name : ";
cin>>rollno>>name;
cout<<"Enter mark1 and mark2 : ";
cin>>mark1>>mark2;
}
void display()
{
cout<<"Rollno = "<<rollno<<endl;
cout<<"Name ="<<name<<endl;
cout<<"Mark1 ="<<mark1<<endl;
cout<<"Mark2 ="<<mark2<<endl;
}
};
main()
{
B b1;
clrscr();
b1.getdata();
b1.display();
return(0);
}
Output
Enter rollno and name : 21
Surjyakanta
Enter mark1 and mark2 :
89
92
Rollno = 21
Name =Surjyakanta
Mark1 =89
Mark2 =92
Explanation
Here the data members of class A are inherited in private mode to class B, so that they cannot be inherited further from class B.
4.2. Multilevel Inheritance
When a single class(B) is derived from a single base class(A), then the inheritance is called as single level inheritance. The mechanism of deriving a class from a derived class is called as multilevel inheritance.
↓
↓
Figure 2 Multilevel Inheritance
Program 2
Write a program to implement multilevel inheritance as per the structure given in figure 3.
#include<iostream.h>
#include<constream.h>
class A
{
protected:
char name[15];
int age;
};
class B: public A
{
protected:
float height;
float weight;
};
class C:private B
{
private:
char game[10];
public:
void getdata()
{
cout<<"Enter name and age : ";
cin>>name>>age;
cout<<"Enter height and weight : ";
cin>>height>>weight;
cout<<"Enter game name :";
cin>>game;
}
void display()
{
cout<<"Name ="<<name<<endl;
cout<<"Age ="<<age<<endl;
cout<<"Height ="<<height<<endl;
cout<<"Weight ="<<weight<<endl;
cout<<"Game ="<<game<<endl;
}
};
main()
{
C c1;
clrscr();
c1.getdata();
c1.display();
return(0);
}
Output
Enter name and age :
Surjyakanta
31
Enter height and weight :
5.5
61
Enter game name :
Cricket
Name =Surjyakanta
Age =31
Height =5.5
Weight =61
Game =Cricket
Explanation
Here the data members of class A are inherited in private mode to class B, so that they cannot be inherited further from class B. Further, the data members of class B are inherited to class C.
4.3. Multiple Inheritances
When a class is derived from more than one base class, then the inheritance is called as multiple inheritances.
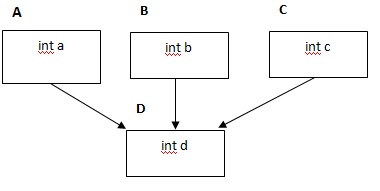
Figure 3 Multiple Inheritances
Program 3
Write a program to implement multiple inheritance as per the structure given in figure 3.
#include<iostream.h>
#include<constream.h>
class A
{
protected:
int a;
};
class B
{
protected:
int b;
};
class C
{
protected:
int c;
};
class D: private A,private B,private C
{
private:
int d;
public:
void getdata()
{
cout<<"Enter values of a,b,c and d :";
cin>>a>>b>>c>>d;
}
void display()
{
cout<<"a ="<<a<<endl;
cout<<"b ="<<b<<endl;
cout<<"c ="<<c<<endl;
cout<<"d ="<<d<<endl;
}
};
main()
{
D d1;
clrscr();
d1.getdata();
d1.display();
return(0);
}
Output
Enter values of a,b,c and d :11
22
33
44
a =11
b =22
c =33
d =44
Explanation
Here the data members of class A,B and C are inherited in private mode to class D, so that they cannot be inherited further from class D.
4.4. Hybrid Inheritance
The combination of more than one type of inheritance is called as hybrid inheritance.
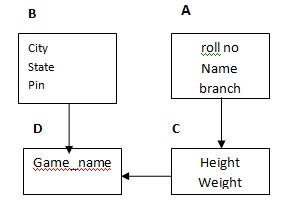
Figure 4 Hybrid Inheritance
Program 4
Write a program to implement hybrid inheritance as per the structure given in figure 4.
#include<iostream.h>
#include<constream.h>
class A
{
protected:
int rollno;
char name[15];
char branch[15];
};
class C
{
protected:
char city[10];
char state[10];
int pin;
};
class B: public A
{
protected:
float height;
float weight;
};
class D: private B, private C
{
private:
char game_name[10];
public:
void getdata()
{
cout<<"Enter rollno, name and branch :";
cin>>rollno>>name>>branch;
cout<<"Enter height and weight :";
cin>>height>>weight;
cout<<"Enter game name :";
cin>>game_name;
}
void display()
{
cout<<"Rollno : "<<rollno<<endl;
cout<<"Name : "<<name<<endl;
cout<<"Branch : "<<branch<<endl;
cout<<"Height : "<<height<<endl;
cout<<"Weight : "<<weight<<endl;
cout<<"Game : "<<game_name<<endl;
}
};
main()
{
D d1;
clrscr();
d1.getdata();
d1.display();
return(0);
}
Output
Enter rollno, name and branch :
21
Surjya
IT
Enter height and weight :
5.5
61
Enter game name :
Cricket
Rollno : 21
Name : Surjya
Branch : IT
Height : 5.5
Weight : 61
Game : Cricket
Explanation
Here the classes A and C are created first. The data members of class A are inherited in protected mode to class B. The data members of both class B and C are inherited to class D in private mode.
4.5. Hierarchical Inheritance
When more than one class is derived from a single base class, then the inheritance is called as hierarchical inheritance.
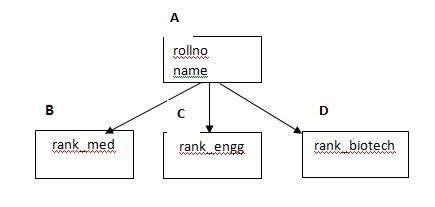
Figure 5. Hierarchical Inheritance
Program 5
Write a program to implement hierarchical inheritance as per the structure given in figure 5.
#include<iostream.h>
#include<constream.h>
class A
{
protected:
int rollno;
char name[15];
};
class B:private A
{
private:
int rank_med;
public:
void getdata()
{
cout<<"Enter rollno and name :";
cin>>rollno>>name;
cout<<"Enter medical rank :";
cin>>rank_med;
}
void display()
{
cout<<"Rollno : "<<rollno<<endl;
cout<<"Name : "<<name<<endl;
cout<<"Medical rank : "<<rank_med<<endl;
}
};
class C:private A
{
private:
int rank_eng;
public:
void getdata()
{
cout<<"Enter rollno and name :";
cin>>rollno>>name;
cout<<"Enter engineering rank :";
cin>>rank_eng;
}
void display()
{
cout<<"Rollno : "<<rollno<<endl;
cout<<"Name : "<<name<<endl;
cout<<"Btech rank : "<<rank_eng<<endl;
}
};
class D:private A
{
private:
int rank_biotech;
public:
void getdata()
{
cout<<"Enter rollno and name :";
cin>>rollno>>name;
cout<<"Enter biotech rank :";
cin>>rank_biotech;
}
void display()
{
cout<<"Rollno : "<<rollno<<endl;
cout<<"Name : "<<name<<endl;
cout<<"Biotech rank : "<<rank_biotech<<endl;
}
};
main()
{
B b1;
C c1;
D d1;
clrscr();
b1.getdata();
b1.display();
c1.getdata();
c1.display();
d1.getdata();
d1.display();
return(0);
}
Output
Enter rollno and name :
21
Manish
Enter medical rank :2345
Rollno : 21
Name : Manish
Medical rank : 2345
Enter rollno and name :
21
Manish
Enter engineering rank :2178
Rollno : 21
Name : Manish
Btech rank : 2178
Enter rollno and name :
21
Manish
Enter biotech rank :3434
Rollno : 21
Name : Manish
Biotech rank : 3434
Explanation
Here the classes A act as a common base class for classes B,C and D respectively. The classes B, C and D are independent of each other as they access the members of class A independently.
5. Virtual Base Class
The virtual base class is a phenom,enon in C++ which is used to avoid the duplication of inherited members in multipath inheritance. We can make the common base class as virtual while declaring the direct or intermediate base class.
The structure of a multipath inheritance is given as:
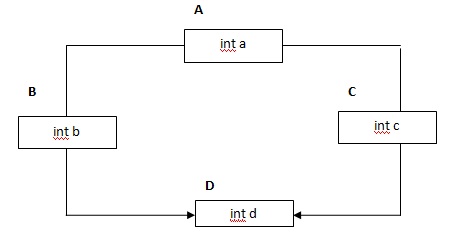
Figure 6.6 Multiple Inheritance
Program 6.6
Write a program to implement multipath inheritance as per the structure given in figure 6.6. Use virtual base class
#include<iostream.h>
#include<constream.h>
class A
{
protected:
int a;
};
class B:public virtual A
{
protected:
int b;
};
class C:virtual public A
{
protected:
int c;
};
class D: private B,private C
{
private:
int d;
public:
void getdata()
{
cout<<"Enter values for a,b,c and d :";
cin>>a>>b>>c>>d;
}
void display()
{
cout<<"a = : "<<a<<endl;
cout<<"b = : "<<b<<endl;
cout<<"c = : "<<c<<endl;
cout<<"d = : "<<d<<endl;
}
};
main()
{
D d1;
clrscr();
d1.getdata();
d1.display();
return(0);
}
Output
Enter values for a, b, c and d :
2
3
4
5
a = : 2
b = : 3
c = : 4
d = : 5
Explanation
The virtual base class is declared while declaring the direct or intermediate base classes B and C with the keyword virtual as :
_________________________
class B:public virtual A
{
protected:
int b;
};
__________________________
class C:virtual public A
{
protected:
int c;
};
____________________________
That means, the keywords virtual and public can be used either of the order as virtual public or public virtual.
Remember
When a class is made as virtual base class, C++ takes necessary step to see that only one copy that particular class is inherited, regardless of how many paths exist between the virtual base class and the derived class.
6. Use of constructors in inheritance
When both base class and derived class contain constructors, the order of execution is summarized in the following Table 2
Table 2
Type of Inheritance
|
Order of Constructor Execution
|
class B: public A
{
//Single Inheritance
}
|
A() ; base constructor first
B() ; derived constructor second
|
class C: public A, public B
{
//Multiple Inheritance
}
|
A() ; 1st base constructor first
B() ; 2nd base constructor second
C() ; 3rd derived constructor third
|
class C: public virtual A, public B
{
//Multiple Inheritance
}
|
A() ; virtual base constructor first
B() ; ordinary base constructor second
C() ; derived constructor third
|
Program 7
Write a program to implement the execution of constructor in single inheritance with arguments.
#include<iostream.h>
#include<constream.h>
class A
{
protected:
int a;
public:
A(int i)
{
a=i;
}
void display1()
{
cout<<"a ="<<a<<endl;
}
};
class B: public A
{
protected:
int b;
public:
B(int x,int y):A(x)
{
b=y;
}
void display2()
{
cout<<"b ="<<b<<endl;
}
};
main()
{
B b1(10,20);
clrscr();
b1.display1();
b1.display2();
return(0);
}
Output
a =10
b =20
Explanation
In the above program, we create an object b1 using the derived class B. In the constructor definition of the derived class B, the value of x is assigned to a in class A, and the value of y is assigned to b of class B.
Program 8
Write a program to implement the execution of constructor in multiple inheritance with arguments.
#include<iostream.h>
#include<constream.h>
class A
{
protected:
int a;
public:
A(int i)
{
a=i;
}
void display1()
{
cout<<"a ="<<a<<endl;
}
};
class B
{
protected:
int b;
public:
B(int j)
{
b=j;
}
void display2()
{
cout<<"b ="<<b<<endl;
}
};
class C: public A,public B
{
protected:
int c;
public:
C(int x,int y,int z):A(x),B(y)
{
c=z;
}
void display3()
{
cout<<"c ="<<c<<endl;
}
};
main()
{
C c1(10,20,30);
clrscr();
c1.display1();
c1.display2();
c1.display3();
return(0);
}
Output
a =10
b =20
c =30
Explanation
In the above program, we create an object c1 using the derived class C. In the constructor definition of the derived class C, the value of x is assigned to a in class A, the value of y is assigned to b of class B, and the value of z is assigned to C of class C.
Summary
1. Inheritance allows us to define a class in terms of another class, which makes it easier to create and maintain an application.
2. It provides an opportunity to reuse the code functionality and fast implementation time.
3. When creating a class, instead of writing completely new data members and member functions, the programmer can designate that the new class should inherit the members of an existing class. This existing class is called the base class, and the new class is referred to as the derived class.
4. The idea of inheritance implements the is a relationship. For example, mammal IS-A animal, dog IS-A mammal hence dog IS-A animal as well and so on.
5. The access-specifier is one of public, protected, or private, and base-class is the name of a previously defined class. If the access-specifier is not used, then it is private by default.
Expertsminds offers Inheritance and Types of Inheritance C++ Assignment Help, Inheritance and C++ Assignment Writing Help, Types of Inheritance Assignment Tutors, Types of Inheritance Solutions, Types of Inheritance Answers, C++ Tutorial Assignment Experts Online